2.1. Python and Jupyterlab#
last update: Feb 07, 2024
Python is a simple and most popular programming language and used in many fields like AI and machine learning, Data analytics, Data visualization, Web, Game, and so on.
JupyterLab is a next-generation web-based user interface for python programming.
Here, you can learn basics of python and usage of JupyterLab. If you have not installed jupyter-lab, refer to venv.
2.1.1. print() function#
print()
function is the most basic function in python. It is used to print the value of any variable or expression.
Here, we will see how to use print()
function with the usage of jupyter lab(notebook).
To begin with, let us print the string “Hello World” using print()
function.
In jupyter lab(notebook), a code block is called a cell
. A cell can contain multiple lines of code.
To execute the code, click on the cell(colored in blue(jupyter notebook:green)) and press Shift+Enter
.
print("Hello, world!")
Hello, world!
comment#
When programming, we often put some comments to explain the code. In python, we can use #
to write comments.
If you want to comment out multiple lines, you can use """ comment """
to write comments (actually, this is called docstrings).
# This is a comment. No output.
"""
This
is
a
multiline
comment.
"""
'\n This\n is\n a \n multiline\n comment.\n'
Arithmetic operations#
print(1+1)
2
In programming, every object has a data type. For example, the number 1
has the data type int
.
The “Hello World” string has the data type str
. The operations will be performed differently depending on the data types of targets.
For example, +
operator is used to add two numbers, and +
operator is used to concatenate two strings.
# you cannot use strings to do math.
print(3+4)
print('3+4')
print('3'+'4')
7
3+4
34
# This will be an error. You can't add a number and a string.
# You need to convert the string to a number or vice versa.
print(3+'4')
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
Cell In[5], line 3
1 # This will be an error. You can't add a number and a string.
2 # You need to convert the string to a number or vice versa.
----> 3 print(3+'4')
TypeError: unsupported operand type(s) for +: 'int' and 'str'
a = 3 # This is a variable.
b = '4'
print(a+b) # This will be the same as the above cell.
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
Cell In[6], line 3
1 a = 3 # This is a variable.
2 b = '4'
----> 3 print(a+b) # This will be the same as the above cell.
TypeError: unsupported operand type(s) for +: 'int' and 'str'
# print out several inputs with `,`
print(a, b)
3 4
print(a + int(b)) # This will convert the string to a number.
print(str(a) + b) # This will convert the number to a string.
7
34
# Basic arithmetic operations
print('addition 1+1 = ', 1+1)
print('subtraction 5-1 = ', 5-1)
print('multiplication 2*2 = ', 2*2)
print('division 5/2 = ', 5/2)
print('modulus 5%2 = ', 5%2)
print('floor division 5//2 = ', 5//2)
print('exponentiation 5**2 = ', 5**2)
addition 1+1 = 2
subtraction 5-1 = 4
multiplication 2*2 = 4
division 5/2 = 2.5
modulus 5%2 = 1
floor division 5//2 = 2
exponentiation 5**2 = 25
# Instead of using a new line, you can use a semicolon.
print('adsf'); print('asdf')
adsf
asdf
jupyter lab usage#
Basically, code cells are used to write code. However, we can also use code cells to write comments in markdown format.
If you want to write in markdown format, right click around the cell and press m
, then Shift+Enter
. You go back to code format by pressing y
.
Moreover, you can add a new cell by pressing a
or b
(a
is for above, b
is for below).
Others; x
; cut a cell. c
; copy a cell. v
; paste a cell (Shift + c
to copy multi cells). z
; undo.
2.1.2. Collections(list, tuple, set, dictionary)#
List, tuple, set, dictionary are used to store data in different ways.
l = [3,4,5]
index = 0 # index starts with 0
print(l[index])
l[1] = 7 # You can change the value of an element in a list.
print(l)
3
[3, 7, 5]
# You can use different types of data in a list.
l = [3,4,5,'a','b','c', True, False, [1,2,3]]
t = (3,4,5)
t[2] = 7
# You can't change the value of an element in a tuple.
# However, a tuple uses less memory than a list.
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
Cell In[13], line 3
1 t = (3,4,5)
----> 3 t[2] = 7
4 # You can't change the value of an element in a tuple.
5 # However, a tuple uses less memory than a list.
TypeError: 'tuple' object does not support item assignment
s = {3,4,5,5}
print(s)
{3, 4, 5}
d = {'a':3,'b':4,'c':5}
print(d['a'])
d['a'] = 6 # You can change the value of an element in a dictionary.
d['d'] = 7 # You can add a new key-value pair to a dictionary.
print(d)
print(d.items()) # This will return a list of all the key-value pairs in the dictionary.
print(d.keys()) # This will return a list of all the keys in the dictionary.
print(d.values()) # This will return a list of all the values in the dictionary.
3
{'a': 6, 'b': 4, 'c': 5, 'd': 7}
dict_items([('a', 6), ('b', 4), ('c', 5), ('d', 7)])
dict_keys(['a', 'b', 'c', 'd'])
dict_values([6, 4, 5, 7])
2.1.3. Open a file#
path = './sample.txt'
with open(path) as f:
l = f.readlines()
print(l)
for i in range(len(l)):
print(l[i])
['One\n', 'Two\n', 'Three\n']
One
Two
Three
2.1.4. For loops#
for loop
is used to iterate over a collection of items.
for i in range(5):
print(i)
# Index starts at 0.
0
1
2
3
4
for i in range(5,10):
print(i)
# Index starts at 5.
# cf. range(5,13,2)
5
6
7
8
9
items = ['a','b','c','d','e']
for i in items:
print(i)
# You can use a for loop to iterate over a list, tuple, dictionary, string, and so on.
print('-------------------------------')
for i in range(len(items)):
print(items[i])
a
b
c
d
e
-------------------------------
a
b
c
d
e
for i, item in enumerate(items):
print(i, item)
0 a
1 b
2 c
3 d
4 e
for _ in range(5):
print('Hello')
Hello
Hello
Hello
Hello
Hello
2.1.5. Comprehension#
[i for i in range(5)]
[0, 1, 2, 3, 4]
data = [3,14,23,28,35,46,55,65,76,87,98,109,120,131,142]
[x*10 for x in data]
[30, 140, 230, 280, 350, 460, 550, 650, 760, 870, 980, 1090, 1200, 1310, 1420]
[x for x in data if x%2==0]
[14, 28, 46, 76, 98, 120, 142]
[x if x>50 else x*10 for x in data]
[30, 140, 230, 280, 350, 460, 55, 65, 76, 87, 98, 109, 120, 131, 142]
You can use list comprehension like below but it is not recommended because it is hard to read.
import numpy as np # This is a module. NumPy is a package that contains many useful functions for working with arrays.
a = np.arange(1,51)
fizz_buzz = ['fizzbuzz' if x%3==0 and x%5==0 else 'fizz' if x%3==0 else 'buzz' if x%5==0 else x for x in a]
print(fizz_buzz)
[1, 2, 'fizz', 4, 'buzz', 'fizz', 7, 8, 'fizz', 'buzz', 11, 'fizz', 13, 14, 'fizzbuzz', 16, 17, 'fizz', 19, 'buzz', 'fizz', 22, 23, 'fizz', 'buzz', 26, 'fizz', 28, 29, 'fizzbuzz', 31, 32, 'fizz', 34, 'buzz', 'fizz', 37, 38, 'fizz', 'buzz', 41, 'fizz', 43, 44, 'fizzbuzz', 46, 47, 'fizz', 49, 'buzz']
2.1.6. Functions#
function
is a block of code which take inputs and returns outputs. It only runs when it is called.
Actually, most code we write consists of functions.
def print_hello():
print('Hello, world!')
print_hello()
Hello, world!
def myfunc(arg1, arg2):
for i in range(arg1):
print(arg2)
myfunc(3, 'Hello')
# = myfunc(arg1=3, arg2='Hello')
Hello
Hello
Hello
import numpy as np # NumPy is a package that contains many useful functions for working with arrays.
import matplotlib.pyplot as plt # Matplotlib is a package that contains many useful functions for plotting.
x = np.linspace(0,10, 100)
y = np.sin(x)
print(x[:5])
print(y[:5])
[0. 0.1010101 0.2020202 0.3030303 0.4040404]
[0. 0.10083842 0.20064886 0.2984138 0.39313661]
plt.plot(x,y)
plt.title('sin')
plt.show()
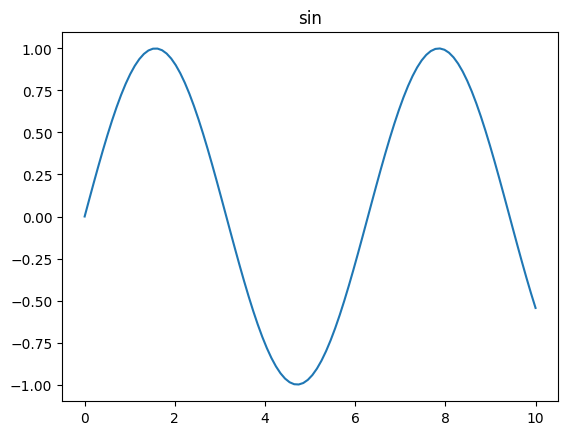
lambda functions#
f = lambda x: x**2
print(f(3))
9